Inputs#
VSPEC
can read in inputs in two ways:
From a YAML file
From a
VSPEC.params.InternalParameters
object
YAML is convenient because it is human-readable and can be easily ported between programs. Creating an InternalParameters
instance directly in Python can also be convenient, especially when running many near-identical models.
The VSPEC.params
YAML Format#
The most basic way to create an ObservationalModel
object instance is through a YAML file:
import VSPEC
model = VSPEC.ObservationModel.from_yaml('my_config.yaml')
The file my_config.yaml
contains all the necessary information to run VSPEC
.
YAML files have a hierarchical structure, so for example the header section looks like this:
header:
data_path: transit
teff_min: 2300 K
teff_max: 3900 K
desc: This is a VSPEC example.
verbose: 0
The sections of a VSPEC
YAML configuration file are below.
Note
Because the configuration file is parsed by a parameter object that knows
what type to expect, it is possible to parse directly to an astropy.units.Quantity
object. Any parameter that expects a quantity will read in the user input as:
u.Quantity(my_input)
So encoding the quantity as a string is very easy. For example the teff_min
parameter can be set to 2300 K
, and so after parsing it will be equivalent
to having set it to 2300 * u.K
.
header
#
Key |
Parses to |
Description |
---|---|---|
|
|
The name of the directory inside |
|
|
The minimum effective temperature to include in the spectrum grid. |
|
|
The maximum effective temperature to include in the spectrum grid. |
|
|
A description of the model. |
|
|
The level of verbosity. |
|
|
The seed for the random number generator. |
|
see spec_grid |
Parameters that define the grid of spectral models. |
spec_grid
#
Key |
Parses to |
Description |
---|---|---|
|
|
The name of the spectral grid. |
Additional parameters (see below) |
Note
The name
parameter determines how the rest of this section is parsed.
See below for additional parameters
name="vspec"
#
This is the default spectral grid what was created for VSPEC. It is made from PHOENIX stellar models [Husser et al., 2013].
Key |
Parses to |
Description |
---|---|---|
|
|
The maximum effective temperature to include in the grid. |
|
|
The minimum effective temperature to include in the grid. |
|
|
The implementation of the binning algorithm, either ‘rust’ or ‘python’. Default is ‘rust’. |
|
|
The implementation of the interpolation algorithm, either ‘scipy’ or ‘jax’. Default is ‘scipy’. |
|
|
If |
name="bb"
#
This replaces the grid interpolator with a forward model that produces a pure blackbody spectrum. This might be useful to use when testing variability as it is very fast.
Note
There are no additional parameters for this spectral model.
star
#
Note
Available presets include static_proxima
, spotted_proxima
, flaring_proxima
, and proxima
.
Key |
Parses to |
Description |
---|---|---|
|
|
The template for the star. See the PSG Handbook for options. |
|
|
The effective temperature of the star. |
|
|
The mass of the star. |
|
|
The radius of the star. |
|
|
The rotational period of the star. |
|
|
The misalignment between the stellar rotation axis and the orbital axis. This is the planet’s “mutual inclination”. |
|
|
The direction of stellar rotation axis misalignment, relative to the argument of periapsis. |
|
See ld |
The limb darkening parameters of the star. |
|
See spots |
The parameters to create star spots. |
|
See faculae |
The parameters to create faculae. |
|
See flares |
The parameters to create flares. |
|
See granulation |
The parameters to create granulation. |
|
|
Stellar surface grid parameters. If |
|
|
The spectral grid to use. Either |
ld
#
Note
Available presets include lambertian
, proxima
, solar
, and trappist
.
Key |
Parses to |
Description |
---|---|---|
|
|
The first limb darkening coefficient. |
|
|
The second limb darkening coefficient. |
spots
#
Note
Available presets include none
, mdwarf
, and solar
.
Key |
Parses to |
Description |
---|---|---|
|
|
The distribution of the spots. Either |
|
|
The spot coverage created initially by generating spots at random stages of life. |
|
|
The fractional coverage of the star’s surface by spots. This is the value at growth-decay equilibrium. |
|
|
The duration of the burn-in period, during which the spot coverage approaches equilibrium. |
|
|
The mean area of a spot on the star’s surface in MSH. |
|
|
The standard deviation of the spot areas. This is a lognormal distribution, so the units of this value are dex. |
|
|
The effective temperature of the spot umbrae. |
|
|
The effective temperature of the spot penumbrae. |
|
|
The rate at which new spots grow. Spots grow exponentially, so this quantity has units of 1/time. |
|
|
The rate at which existing spots decay [area/time]. |
|
|
The area of a spot at birth. |
faculae
#
Note
Available presets include none
and std
.
Key |
Parses to |
Description |
---|---|---|
|
|
The distribution of the spots. Currently only |
|
|
The fractional coverage of the star’s surface by spots. This is the value at growth-decay equilibrium. |
|
|
The duration of the burn-in period, during which the spot coverage approaches equilibrium. |
|
|
The mean radius of the faculae. |
|
|
The standard deviation of the radius in dex. |
|
|
The mean faculae lifetime. |
|
|
The standard deviation of the lifetime in dex. |
|
|
The depth of the facula depression. |
|
|
The slope of the radius-Teff relationship for the cool floor. |
|
|
The minimum radius at which the floor is visible. Otherwise the facula is a bright point – even near disk center. |
|
|
The Teff of the floor at the minimum radius. |
|
|
The slope of the radius-Teff relationship for the hot wall. |
|
|
The intercept of the radius-Teff relationship for the hot wall. |
Note
The temperatures of a facula wall or floor are a function of the radius. See the vspec-vsm source for information on how these values are calculated.
flares
#
Note
Available presets include none
and std
.
Key |
Parses to |
Description |
---|---|---|
|
|
The mean temperature of the flares. |
|
|
The standard deviation of the flare temperatures. |
|
|
The mean FWHM of the flare lightcurves [time]. |
|
|
The standard deviation of the FWHM in dex. |
|
|
The slope of the frequency-energy powerlaw. |
|
|
The intercept of the frequency-energy powerlaw. |
|
|
The minimum energy of the flares. Set to infinity to disable. |
|
|
The typical number of flares in each cluster. |
granulation
#
Note
Available presets include none
and std
.
Key |
Parses to |
Description |
---|---|---|
|
|
The mean coverage of low-teff granulation. |
|
|
The amplitude of granulation oscillations. |
|
|
The period of granulation oscillations. |
|
|
The difference between the quiet photosphere and the low-teff granulation region. |
planet
#
Note
Available presets include proxcenb
and std
.
Key |
Parses to |
Description |
---|---|---|
|
|
The name of the planet. |
|
|
The radius of the planet. |
|
see gravity |
The mass/surface gravity/density of the planet. |
|
|
The semi-major axis of the planet’s orbit. |
|
|
The period of the planet’s orbit. |
|
|
The rotation period of the planet. |
|
|
The eccentricity of the planet’s orbit |
|
|
The obliquity (tilt) of the planet. Not currently implemented. |
|
|
The direction of the planet’s obliquity. The true anomaly at which the planet’s north pole faces away from the star. |
|
|
The phase of the planet at the beginning of the simulation. |
|
|
The initial substellar longitude of the planet. |
gravity
#
Key |
Parses to |
Description |
---|---|---|
|
|
The mode of the gravity parameter. Valid options are ‘g’, ‘rho’, and ‘kg’. |
|
|
The value of the gravity parameter. Can be any unit so long as the physical type is correct. |
system
#
Key |
Parses to |
Description |
---|---|---|
|
|
The distance to the system. |
|
|
The inclination of the system. Transit occurs when \(i=90^{\circ}\). |
|
|
The phase of the planet when it reaches periasteron. Only necessary for planets with non-zero obliquity or non-zero eccentricity. |
obs
#
Key |
Parses to |
Description |
---|---|---|
|
|
The total time of the observation. |
|
|
The integration time of each epoch of observation. |
inst
#
Note
Available presets include mirecle
, miri_lrs
, and niriss_soss
.
Key |
Parses to |
Description |
---|---|---|
|
see single or coronagraph |
The type of telescope to use. |
|
see bandpass |
The bandpass & resolution of the observation. |
|
see detector |
The detector properties of the observation. |
single
#
Key |
Parses to |
Description |
---|---|---|
|
|
The aperture size of the telescope. |
|
|
The level of the zodiacal background. See the PSG Handbook for details. |
coronagraph
#
Warning
Use this instrument type with caution. It is not well tested and not fully implemented. Pull requests are welcome.
Key |
Parses to |
Description |
---|---|---|
|
|
The aperture size of the telescope. |
|
|
The level of the zodiacal background. See the PSG Handbook for details. |
|
|
The contrast of the coronagraphic system. |
|
|
The inner working angle of the coronagraph. |
bandpass
#
Key |
Parses to |
Description |
---|---|---|
|
|
The minimum wavelength. |
|
|
The maximum wavelength. |
|
|
The resolving power of the observation. |
|
|
The unit to be used on the spectral axis. |
|
|
The unit to be used on the flux axis. |
detector
#
Key |
Parses to |
Description |
---|---|---|
|
|
The width of the field of view. |
|
|
The integration time on the detector for saturation purposes. This effects the read noise of the observation. |
|
see ccd |
The CCD used for the observation. |
ccd
#
Key |
Parses to |
Description |
---|---|---|
|
|
The number of pixels comprising a resolution element. |
|
|
The read noise of the CCD in electrons. |
|
|
The dark current of the CCD in electrons/second. |
|
|
The throughput of the optical system. |
|
|
The emissivity of the optical system. |
|
|
The temperature of the optical system. |
psg
#
Key |
Parses to |
Description |
---|---|---|
|
|
The number of GCM points to bin together. Use 200 for testing and 3 for science (depending on the native resolution of your gcm). |
|
|
Planetary epoch binning. Useful if your star changes on a shorter timescale than your planet. For example, if set to 4, a planet spectrum will be calculated for every fourth stellar spectrum and will be interpolated otherwise. |
|
|
Whether to have PSG consider the planetary atmosphere. If False, PSG will return a blackbody spectrum. Useful for testing. |
|
|
Whether to have PSG use a stellar atmosphere model. Otherwise, the stellar spectrum is a blackbody.
While not guaranteed, there is no expectation that this parameter has any effect on VSPEC outputs.
Default is |
|
|
The number of n-stream pairs for scattering aerosols calculations. See the PSG Handbook for details. |
|
|
The number of Legendre polynomials for scattering aerosols calculations. See the PSG Handbook for details. |
|
|
The continuum opacities to include in the radiative transfer calculation. Typically these include
|
gcm
#
Note
In order to support many types of GCM input this section can be configured many ways.Read the following carefully.
Key |
Parses to |
Description |
---|---|---|
|
|
The mean molecular weight of the GCM in amu. |
|
The GCM type and parameters. |
binary
#
Key |
Parses to |
Description |
---|---|---|
|
|
The path to the PSG-readable GCM file. The contents of this file are parsed
by |
waccm
#
Key |
Parses to |
Description |
---|---|---|
|
|
The path to the WACCM netCDF file. |
|
|
The index of the time axis to use. If this is specified, then the GCM is static. Otherwise,
it is assumed that the GCM changes as a function of time, and the |
|
|
The start time of the GCM. Only used if |
|
|
Which molecular species to look for in the GCM file. Use the same names as in PSG. |
|
|
Which aerosol species to look for in the GCM file. Use the same names as in PSG. |
|
|
The background molecular species. The abundances in |
|
|
The longitude of the first point on the longitudinal axis. Default is |
|
|
The latitude of the first point on the latitudinal axis. Default is |
exocam
#
Key |
Parses to |
Description |
---|---|---|
|
|
The path to the ExoCAM netCDF file. |
|
|
The index of the time axis to use. If this is specified, then the GCM is static. Otherwise,
it is assumed that the GCM changes as a function of time, and the |
|
|
The start time of the GCM. Only used if |
|
|
Which molecular species to look for in the GCM file. Use the same names as in PSG. |
|
|
Which aerosol species to look for in the GCM file. Use the same names as in PSG. |
|
|
The background molecular species. The abundances in |
|
|
The longitude of the first point on the longitudinal axis. Default is |
|
|
The latitude of the first point on the latitudinal axis. Default is |
|
|
The mean molecular mass of the GCM. Must be specified if |
exoplasim
#
Key |
Parses to |
Description |
---|---|---|
|
|
The path to the ExoPlasim netCDF file. |
|
|
The index of the time axis to use. |
|
|
Which molecular species to look for in the GCM file. Use the same names as in PSG. |
|
|
Which aerosol species to look for in the GCM file. Use the same names as in PSG. |
|
|
The background molecular species. The abundances in |
|
|
The longitude of the first point on the longitudinal axis. Default is |
|
|
The latitude of the first point on the latitudinal axis. Default is |
|
|
The mean molecular mass of the GCM. Must be specified if |
vspec
#
Warning
This is a minimal atmosphere model. It is useful for testing or when a sophisticated model is not necessary.
Key |
Parses to |
Description |
---|---|---|
|
|
The number of layers in the GCM. |
|
|
The number of longitude points in the GCM. |
|
|
The number of latitude points in the GCM. |
|
|
The thermal inertia of the GCM. See Cowan and Agol [2011]. |
|
|
The surface pressure of the GCM. |
|
|
The pressure at the top of the atmosphere. |
|
|
The U component (west-to-east) of the GCM’s wind. |
|
|
The V component (south-to-north) of the GCM’s wind. |
|
|
The adiabatic index of the GCM atmosphere. |
|
|
The surface albedo of the GCM. |
|
|
The surface emissivity of the GCM. |
|
|
The molecular species in the GCM. |
|
|
The latitudinal redistribution factor of the GCM. If |
VSPEC.params Package#
The VSPEC
parameters module.
This classes instruct the behavior of the rest
of the VSPEC
package.
Classes#
|
Class to store bandpass parameters for observations. |
|
Base class for Parameters |
Parameter container for a forward-model blackbody stellar spectrum. |
|
|
Parameters for a coronagraph telescope. |
|
Class to store detector parameters for observations. |
|
Facula Parameters |
|
Class to store stellar flare parameters |
|
Granulation Parameters |
|
Class representing gravity parameters. |
|
Header for VSPEC simulation |
|
Class to store instrument parameters for observations. |
|
Class to store parameters for a VSPEC simulation. |
|
Limb Darkening Parameters for the Quadratic Limb Darkening Law |
|
Class storing parameters for observations. |
|
Class representing planet parameters. |
|
Parameters for a single dish telescope. |
|
Parameters controling variability from star spots. |
|
Parameters describing the |
|
Class representing system parameters. |
|
Base class for telescope Parameters. |
|
Parameter container for the default VSPEC grid. |
|
Class to store CCD parameters for observations. |
|
Class to store GCM parameters. |
|
Class to store parameters for the Planetary Spectrum Generator (PSG). |
Class Inheritance Diagram#
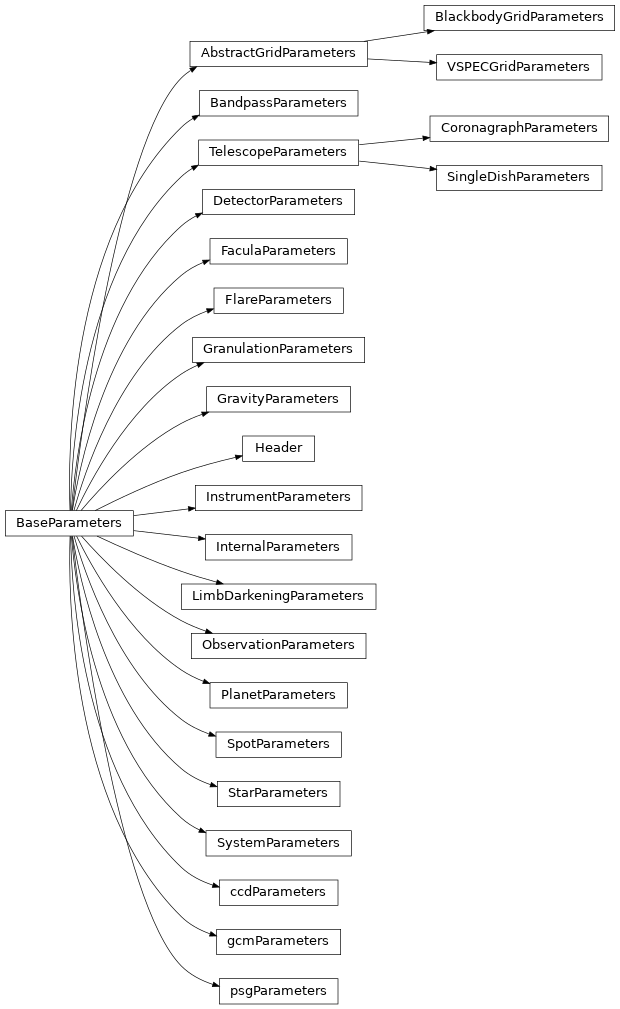